Helika Web SDK (Latest)
Top of the funnel fingerprinting of your community upon first click.
Helika Web SDK Docs
The Helika SDK is a powerful toolkit for integrating Helika services into your applications. Implementing the Helika SDK, enables the ability to leverage the full capabilities of Helika and build robust and scalable solutions.
Features
- Streamline integration with Helika services
- Access to a wide range of Helika APIs
Current Version: 0.3.2
Installation
To install the Helika SDK, you can use npm:
npm install helika-sdk
Quick Start
You’ll need a Helika API Key in order to instantiate the Helika SDK. Please reach out to your Client Success Manager in order to receive an API key for your organization.
Here's a quick example on how to get started with the Helika SDK:
import Helika, { DisableDataSettings, EventsBaseURL } from "helika-sdk"
var helikaSDK;
try {
// Initialize the Helika SDK - it will throw if you use an invalid API key
helikaSDK = new Helika.EVENTS(
'YOUR_API_KEY',
'YOUR_GAME_ID', // alphanumeric, only underscore, no spaces,
EventsBaseURL.EVENTS_DEV
);
} catch (e) {
console.error("api key is invalid");
}
// Optional - if you want to disable Personal Identifiable Information Tracking due to compliance
await helikaSDK.setPIITracking(false);
// Set the default App Details. These will be appended to all events.
helikaSDK.setAppDetails({
platform_id: 'android', // Optional - android, ios, web, desktop, etc.
client_app_version: '1.0.0', // optional - only if it's a client app
server_app_version: '1.0.0', // optional - set only if the sdk is installed on the server
store_id: 'steam', // optional
source_id: 'facebook', // optional
})
// Include any user identifying information that you'd like to keep track of such as any
// emails, wallet addresses, player_id, group_id, usernames, etc.
helikaSDK.setUserDetails({
user_id: '123456',
email: '[email protected]',
wallet_id: '0x4kd....'
})
// Start a session/create a new session which initiates the SDK instance with a
// sessionId which is required to fire events. This should only be called when
// the user lands on the page. We store and re-use the session_id from the local
// storage, if present.
await helikaSDK.startSession();
// Instantiate events based on your game's event taxonomy
const events = [
{
event_type: 'user_register', // required
event: { // required
event_sub_type: 'register', // required
event_details: {
// Client defined information
event_label: "event_label",
event_action: "event_action"
},
win_number: 1,
user_details: {
discord_id: "some_discord_id", // you can optionally add custom user_details information
twitter_id: 'twitterid'
}
}
},
{
event_type: 'user_download', // required
event: { // required
event_sub_type: 'download',
event_details: {
// Client defined information
event_label: "event_label", // Type: varchar
event_action: "event_action" // # Type: varchar
},
timestamp: 12391989
}
}
];
// Asynchronously
// await helika.createEvent(events);
// Send an Event with User Details attached to it
helikaSDK.createUserEvent(events)
.then(response => {
console.log(response);
})
.catch(error => {
console.error(error);
});
// Send an Event to Helika (no user details, user_id is set to anon_id)
helikaSDK.createEvent(events)
.then(response => {
console.log(response);
})
.catch(error => {
console.error(error);
});
createEvent()
vscreateUserEvent()
createEvent()
andcreateUserEvent()
are roughly the same in that they send events to the Helika Service along with appended data.createUserEvent()
, however, appends the user_details object set bysetUserDetails()
whereascreateEvent()
does not. theuser_id
field increateEvent()
also gets replaced by an anonymous id.Please make sure to use the correct create event function that suits the your needs.
Upgrading from 0.2.x
There are significant differences between 0.2.x and 0.3.x. The following functions should be called before
helikaSDK.startSession()
is called:
async helikaSDK.setPIITracking()
helikaSDK.setAppDetails({ platform_id: 'android', // Optional - android, ios, web, desktop, etc. client_app_version: '1.0.0', // optional - only if it's a client app server_app_version: '1.0.0', // optional - set only if the sdk is installed on the server store_id: 'steam', // optional source_id: 'facebook', // optional })
helikaSDK.setUserDetails({ user_id: '123456', email: '[[email protected]](mailto:[email protected])', wallet_id: '0x4kd....' })
- Replace any
get/setPlayerId()
withget/setUserDetails()
- Remove
helikaSDK.setDataSettings()
calls (if used)- Remove
helikaSDK.createUAEvent()
calls (if used)The 0.3.x version now implements our new standard Event Taxonomy. Please refer to:
API Reference
Conventions
The EventsBaseURL is an enum that dictates where to send all API requests. The SDK will handle the set-up for you. You just need to provide an API key and select whether it’s for production
or development
.
For testing purposes, we've added EVENTS_LOCAL
which logs your events to console.log() instead of sending the events.
enum EventsBaseURL {
EVENTS_LOCAL,
EVENTS_DEV,
EVENTS_PROD
}
Game Event Taxonomy
In order for our event processors to parse your events, they must adhere to the set guidelines below.
Example Event
{
"event_type": "register",
"event": {
"event_sub_type": "user_register",
"event_details": {
"event_label": "event_label",
"event_action": "event_action"
},
// Custom User details that weren't set with the default SetUserDetails()
"user_details": {
"discord_id": "some_discord_id"
}
// Other details
"win_number": 1,
}
}
Default Data
App Details
The developer can set specific app details. helikaSDK.setAppDetails()
adds default details for the app which will be included with all events. All of these are optional, but setting them enables better data quality and better analytics.
Field | Type | Required/Optional | Description |
---|---|---|---|
platform_id | string | optional | Specifies the platform (e.g., 'android', 'ios', 'web', etc.). |
client_app_version | string | optional | Version of the client-side app (optional). |
server_app_version | string | optional | Version of the server-side app (optional). |
store_id | string | optional | The distribution store (optional). |
source_id | string | optional | The source of the user (optional, e.g., 'facebook'). |
User Details
When a user logs in, the developer can set the user-specific details using helikaSDK.setUserDetails
. The SDK automatically appends this information to the event in the user_details
field. Adding more information enables better quality data and better analytics.
If a user does not authenticate and no user_id is sent to Helika, an Anonymous ID will be created on session_create
allowing the required field to be satisfied. When the User Details is finally set, after authentication, Helika will associate the Anonymous ID with this authenticated user to provide consistency relating events back to a single user.
Field | Type | Required/Optional | Description |
---|---|---|---|
user_id | string | required | A client defined unique identifier for the user. |
string | optional | The user's email address. | |
wallet_id | string | optional | A cryptocurrency wallet address, if applicable. |
Personal Identifiable Information
The developer has the ability to disable PII (Personal Identifiable Information) tracking using async helikaSDK.setPIITracking(false)
in order to comply with privacy regulations. This needs to get set before the StartSession()
because the PII will be added or omitted in the session_created
event.
If, during the session, the user decides to enable PII tracking, the developer can turn on PII Tracking and it will fire an event to grab PII.
Automatic Data Capture
The Helika SDK automatically appends some data fields in the event along side your customized data event.
Field | Type | Required/Optional | Description |
---|---|---|---|
game_id | string | required | The Game Identifier attribute for all events generated for this game |
created_at | DateTime | required | The timestamp when the event was created. |
session_id | u4 | required | The sdk generates a new Session ID when the Helika Object is created. |
utms | object | optional | The SDK parses the url parameters and records any important or interesting UTMs. |
helika_referral_link | string | optional | The Referral Link ID of the link that the user clicked in order to get to the game’s landing page. |
user_details | object | required | The user details set by 'setUserDetails' |
app_details | object | required | The app details set by 'setAppDetails' |
Clearing Local Storage
We store some saved information such as session_id and the user's anon_id in local storage. Clearing the Local Storage will reset this information and cause new information to be generated.
Methods
Name | URL | Parameter | Description |
---|---|---|---|
constructor | Events() | api_key: string, game_id: string, baseURL: EventsBaseURL | Constructs the Event Service |
Start Session | Events.startSession() | This starts a user session, stores identifying user information, and UTMs that will get appended to events. | |
Create Event | Events.createEvent() | events: { game_id: string, event_type: string, event: Object }[] | Sends the events to the Helika services. This does not append the user_details info to the event and sets 'user_id' to the anonymous_id |
Create User Event | Events.createUserEvent() | events: { game_id: string, event_type: string, event: Object }[] | Sends the events with user information to the Helika services. This appends the user_details object set by setUserDetails() |
Get PII Tracking | Events.getPIITracking | Returns the value of PII Tracking | |
Set PII Tracking | Events.setPIITracking() | piiTracking: boolean | This function disables tracking of personal identifiable information (PII) to comply with privacy regulations. |
Get App Details | Events.getAppDetails() | Obtainsthe currently set app_details | |
Set App Details | Events.setAppDetails() | details: Object | Sets the app_details field that gets added to all events |
Get User Details | Events.getUserDetails | Obtains the currently set user_details | |
Set User Details | Events.setUserDetails() | details: Object | Gets the user_details that will get appended to all events |
Set Enabled | Events.setEnabled() | enabled: boolean | Sets whether it's sending the events to our data pipeline or simply logging the event to console.log(). This is used for testing purposes. |
Is Enabled | Events.isEnabled() | Gets whether the Sending Events is enabled or disabled |
Validation
It is recommended that your team verifies the events are being ingested correctly by sending a series of test events via the SDK. Successfully sent events should have a 200
status response.
Check within your portal for the events to appear in the Data Ingestion Summary.
The Data Ingestion Summary report can be found in the side menu:
Data Summary > Data Ingestion Summary
The Data Ingestion Summary will display information such as:
- Which environment the events are being received in
- Total Event Volumes
- Types, and Sub Types Seen
- A table for the raw event stream, with raw payload.
Ingestion Delays
Please expect up to the following delays during event processing, before results are populated:
- Production: 15 minutes
- Development: 2 hours
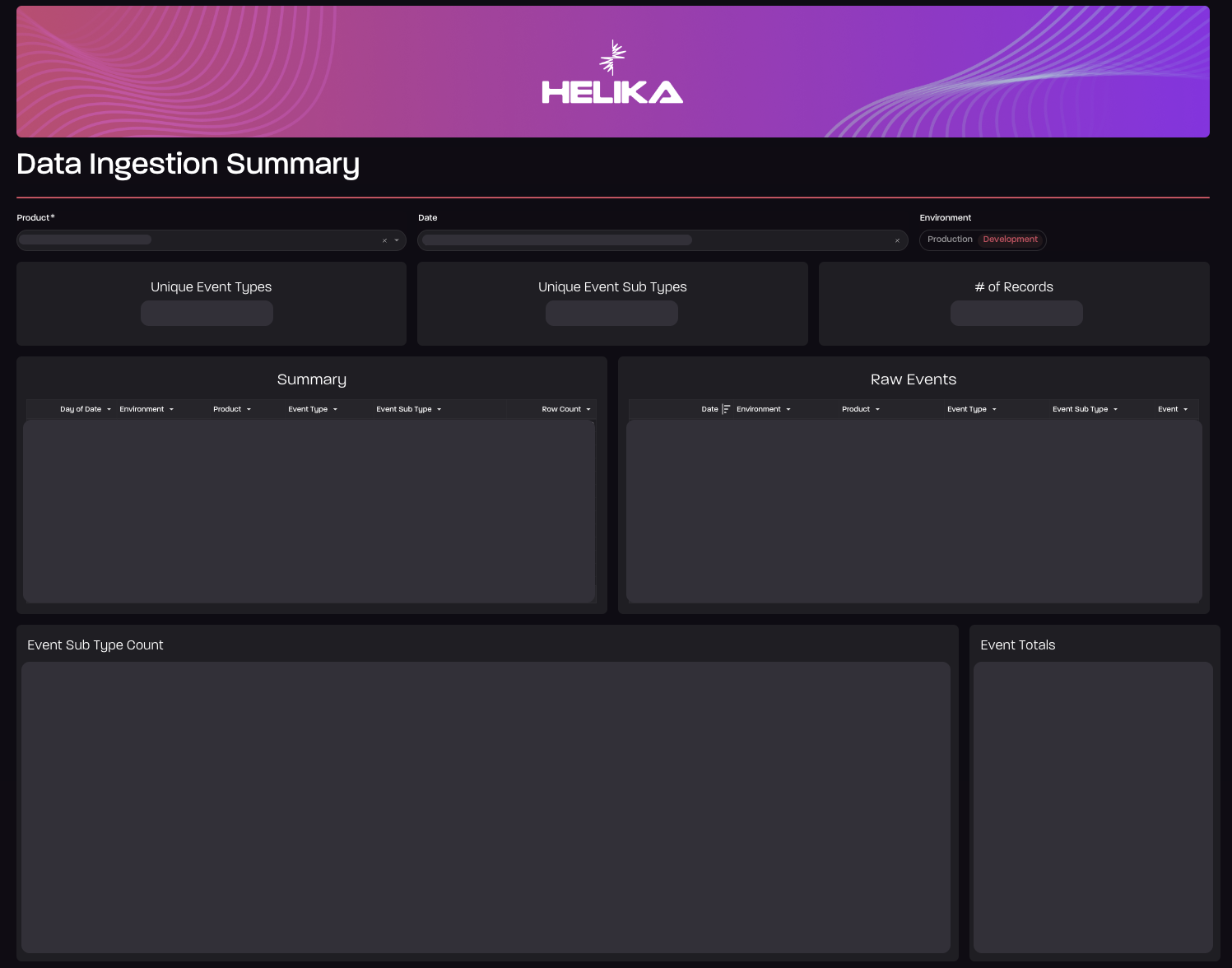
After confirming test events appear in the data summary table, this report can be used to monitor your ongoing events and event volumes, as they are added.
License
The Helika SDK is released under the MIT License.
Updated 5 months ago